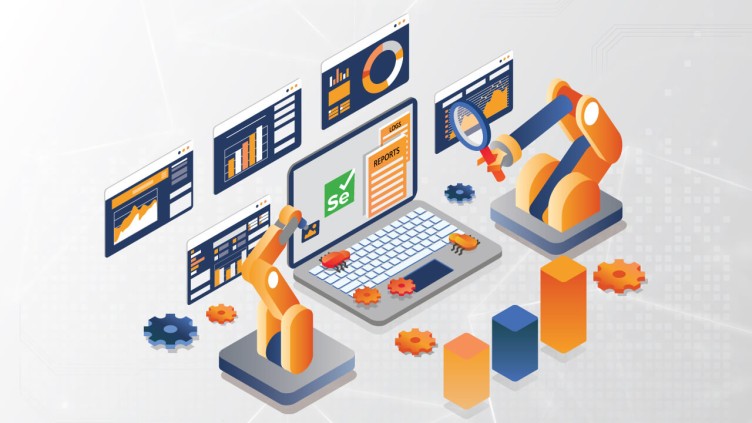
Selenium automation testing plays a crucial role in expediting feedback and catching glitches at the early stages of development. It’s also a game-changer when it comes to cutting down the time and expenses associated with manual testing by handling repetitive tasks through automation. Thanks to its impressive capabilities and adaptability, Selenium has solidified its position as the tool of choice for developers and testers across the industry.
Selenium’s versatility extends to its ability to automate a range of testing tasks in a production environment. Moreover, it serves as a robust development tool, enabling testing even in scenarios where applications are not yet fully developed. This adaptability and effectiveness make Selenium a valuable asset in the pursuit of high-quality software development.
In this article, we’ll dig into some handy Selenium tips and tricks aimed at mastering your testing skills. These valuable insights will not only elevate your Selenium testing proficiency but also bolster the reliability and resilience of your tests.
Why Choose Selenium?
Selenium is a standout choice when it comes to automated testing tools. It’s renowned as one of the most widely used open-source solutions, designed to simplify web browser testing by enabling the creation of automation scripts. The allure of Selenium lies not only in its popularity but also in the extensive online community support it enjoys.
What truly sets Selenium apart is its remarkable versatility. It possesses the capability to conduct automated tests across a range of web browsers, encompassing Chrome, Firefox, Opera, and Internet Explorer. Furthermore, Selenium offers compatibility with various programming languages, including Java, C#, Ruby, and Python. This adaptability makes Selenium an appealing option, particularly for teams that boast developers proficient in different coding languages.
Tips and Tricks for Selenium Automation Testing
Trick #1 – Running Multiple Tests with the Same Parameters
This technique is handy for browser automation scenarios where you need to reuse the same values across different tests. Leveraging TestNG’s capability, you can define specific parameters for a test suite in the configuration file.
In the following example, the code is designed to provide the URL for the WebDriver method to execute a test suite within the TestNG framework.
“`
public class TestNGParameterExample {
@Parameters({“firstSampleParameter”})
@Test(enabled = true)
public void firstTestForOneParameter(String firstSampleParameter) {
driver.get(firstSampleParameter);
WebElement searchBar = driver.findElement(By.linkText(“Mobile Device & Browser Lab”));
searchBar.click();
Assert.assertEquals(driver.findElement(By.tagName(“h1”)).getText(), “Mobile Device & Browser Lab”);
}
@Parameters({“firstSampleParameter”})
@Test
public void secondTestForOneParameter(String firstSampleParameter) {
driver.get(firstSampleParameter);
WebElement searchBar = driver.findElement(By.linkText(“Live Cross Browser Testing”));
searchBar.click();
Assert.assertEquals(driver.findElement(By.tagName(“h1”)).getText(), “Cross Browser Testing”);
}
}
“`
The structure of the `testng.xml` file is illustrated below:
“`
<!DOCTYPE suite SYSTEM “https://testng.org/testng-1.0.dtd” >
<suite name=”Suite1″ verbose=”1″ >
<parameter name=”firstSampleParameter” value=”https://experitest.com/”/>
<test name=”Nopackage” >
<classes>
<class name=”TestNGParameterExample” />
</classes>
</test>
</suite>
“`
This approach allows you to efficiently manage tests with shared parameters, enhancing the reusability and maintainability of your automation suite.
Trick #2 – Dealing with Pop-Up Windows
A common challenge in Selenium web automation is handling pop-up windows, which typically come in three forms:
- Simple alert: displaying a message.
- Confirmation alert: asking the user to confirm an action.
- Prompt alert: requesting user input.
While WebDriver can’t manage Windows-based alerts, it can handle web-based ones. You can use the `switch_to` method to work with these pop-ups.
Here’s an example of a web page with a simple alert:
“`
<!DOCTYPE html>
<html>
<body>
<h2>
Demo for Alert</h3>
Clicking below button
<button onclick=”create_alert_dialogue()” name =”submit”>Alert Creation</button>
<script>
function create_alert_dialogue() {
alert(“Simple alert, please click OK to accept”);
}
</script>
</body>
</html>
“`
In this instance, we use the `switch_to` method to interact with the alert pop-up. Once switched to the alert, we accept it with `alert.accept()`.
“`
from selenium import webdriver
import time
driver = webdriver.Firefox()
driver.get(“file://<HTML File location>”)
driver.find_element_by_name(“submit”).click()
time.sleep(5)
alert = driver.switch_to.alert
text_in_alert = alert.text
print(“The alert message is ” + text_in_alert)
time.sleep(10)
alert.accept()
print(“Alert test is complete”)
driver.close()
“`
This approach enables you to effectively handle web-based pop-ups in your Selenium automation, making your testing more robust and user-friendly.
Trick #3 – Handling Timeouts and Browser Crashes with WebDriverWait
WebDriverWait is a nifty tool in web automation that helps you manage timeouts and browser crashes effectively. Here’s how you can use WebDriverWait:
- Add Dependency: First, make sure you’ve added the necessary dependency to your project. This dependency ensures you have access to WebDriverWait.
“`
<!– https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java –>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.6.0</version>
</dependency>
“`
- Import Libraries: Import the required libraries in your code.
“`
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.*; // This is the WebDriverWait library.
“`
- Create WebDriverWait Instance: Instantiate a new WebDriverWait object, specifying the WebDriver and the timeout duration in seconds.
“`
WebDriver driver = new ChromeDriver();
WebDriverWait wait = new WebDriverWait(driver, 10); // Here, “10” is the timeout in seconds.
“`
- Define a Condition to Wait For: Specify the condition you want to wait for. In this example, we’re waiting for an element with the ID “my-element” to become visible on the page.
“`
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“my-element”)));
“`
This code will patiently wait for up to 10 seconds for the element with the ID “my-element” to appear on the page. If it doesn’t show up within that time frame, WebDriver will throw an error, letting you know that something isn’t as expected.
Using WebDriverWait in your Selenium tests can help you gracefully handle situations where elements take some time to load or when you encounter unexpected delays due to browser issues.
Trick #4 – Recording and Running Tests with Selenium IDE
Selenium IDE is a handy Firefox browser plugin that simplifies the process of recording and running tests. It’s an excellent starting point for beginners in Selenium automation. Here’s how you can record and playback tests using Selenium IDE:
Recording a Test with Selenium IDE:
- Open your Firefox browser and install the Selenium IDE plugin.
- Launch the Selenium IDE tool.
- Click the “New” button to create a new test.
- Give your test a meaningful name for easy identification.
- Click the “Record” button in Selenium IDE, and it will start capturing your interactions with the website.
- Perform the actions you want to include in your test on the target website.
- Once you’ve completed your test scenario, click the “Stop” button in Selenium IDE to finish the recording.
Playing Back a Recorded Test with Selenium IDE:
- Open your Firefox browser and ensure you have the Selenium IDE plugin installed.
- Launch the Selenium IDE tool.
- Click the “Open” button within Selenium IDE, and select the previously recorded test you want to play back.
- With your test loaded, click the “Play” button, and Selenium IDE will execute the recorded steps on the website.
That’s it! Selenium IDE simplifies test automation by allowing you to create and replay tests without the need to write code. It’s a valuable tool for both newcomers and experienced testers looking for quick and straightforward test automation.
Trick #5 – Enhance Test Clarity with Annotations
Annotations serve as a valuable tool to enhance the clarity and organization of your Selenium tests. In Java, annotations are special classes that allow you to add metadata to your code, making it easier to understand and maintain. Think of them as helpful labels for your code. Here are a few essential annotations you can use:
- @Test: This annotation marks a method as a test method. It’s a clear indicator that a particular method is meant for testing a specific aspect of your application.
- @BeforeClass: Use this annotation when you want a method to run before the first test in a class begins. It’s useful for setting up tasks that are common to all tests in that class.
- @AfterClass: Conversely, this annotation runs a method after the last test in a class is completed. It’s handy for performing cleanup or teardown tasks.
- @Before: When you need a method to run before each individual test, use the @Before annotation. It’s perfect for setting up the test environment.
- @After: Similarly, the @After annotation runs a method after each test. It’s useful for cleaning up resources or resetting conditions.
By judiciously applying these annotations, you can significantly improve the organization and comprehensibility of your Selenium test suite. Annotations act as signposts, making it clear how each method contributes to your testing efforts.
Trick #6 – Use Custom Assertions to Verify Data
One of the key aspects of Selenium test automation is understanding assertions and how to use them effectively to validate the behavior of your web applications. Selenium provides built-in assertions for data validation, but there are instances when you need to create custom assertions tailored to your application’s specific needs. Here’s a step-by-step guide on how to create and use custom assertions:
- Create a Java Class: Begin by creating a Java class that extends the Selenium Assert class. This class will serve as the foundation for your custom assertions.
- Add a Method: In your custom assertion class, add a method that accepts two parameters. The first parameter should be an object representing the data you want to validate, and the second parameter is a message to be displayed in case the validation fails.
- Implement Your Logic: Within the method you’ve created, implement the logic for your custom assertion. This logic should define how the data validation should occur.
- Register Your Custom Assertion: To make your custom assertion available for use in your tests, register it with Selenium. You can do this by adding a line of code like this to your test script:
“`
Assert.registerCustomAssertion(new MyCustomAssertion());
“`
Here, “MyCustomAssertion()” should be replaced with the name of your custom assertion class.
- Use Your Custom Assertion: Finally, you can utilize your custom assertion in your test scripts. Call the method you created in your custom assertion class to validate the specific data in your application.
In summary, while Selenium offers a robust set of built-in assertions, creating custom assertions empowers you to tailor your validation to your application’s unique requirements. This flexibility ensures that your automated tests are thorough and align with the specific behavior of your web application.
Trick #7 – Passing Basic Values to Tests
In this scenario, the aim is to create tests that can handle various sets of data efficiently. To achieve this, we make use of the DataProvider method, which has the ability to return a two-dimensional array of objects.
- The first dimension of the array signifies the number of test executions.
- The second dimension corresponds to the count and types of test values.
To gain a better understanding, let’s examine the code example below that employs the @DataProvider annotation:
“`
@DataProvider()
public Object[][] listOfLinks() {
return new Object[][] {
{“Mobile Device & Browser Lab”, “Mobile Device & Browser Lab”},
{“Live Cross Browser Testing”, “Cross Browser Testing”},
{“Automated Mobile Testing”, “Mobile Test Automation!”},
};
}
“`
In the code snippet above, we’ve defined a data provider method named `listOfLinks`. It returns a two-dimensional array with pairs of values. Each pair consists of a link name and its corresponding header.
Now, let’s look at how this data provider is used in a test method:
“`
@Test(dataProvider = “listOfLinks”)
public void firstTestWithSimpleData(String linkName, String header) {
driver.get(“https://experitest.com/”);
WebElement searchBar = driver.findElement(By.linkText(linkName));
searchBar.click();
Assert.assertEquals(driver.findElement(By.tagName(“h1”)).getText(), header);
}
“
In this test method, we specify `@Test(dataProvider = “listOfLinks”)` to indicate that it should use the data provided by the `listOfLinks` data provider. The test method takes two parameters, `linkName` and `header`, which correspond to the values provided by the data provider.
This approach allows us to efficiently conduct tests with various data combinations, enhancing the flexibility and coverage of our automated tests.
Now, if you want to extend your test coverage to different browsers and platforms, you can integrate LambdaTest into your testing process. LambdaTest is an AI-powered test orchestration and test execution platform that allows you to run your Selenium automation tests on a wide range of browsers and operating systems simultaneously. By seamlessly integrating LambdaTest with popular test automation testing frameworks like TestNG or JUnit, you can easily execute your tests in parallel, significantly reducing test execution time and ensuring compatibility across various environments.
With LambdaTest, you can effortlessly scale your Selenium automation testing efforts and enhance your test coverage, ensuring your web application performs flawlessly across different browser and platform combinations.
Including LambdaTest in your Selenium automation testing toolkit opens up opportunities for comprehensive cross-browser and cross-platform testing, helping you deliver a top-notch user experience to all your users, regardless of their choice of browser or device.
Some Additional Valuable Tips and Tricks for Effective Selenium Automation Testing
Here are some additional valuable tips and tricks for effective Selenium automation testing:
- Utilize Explicit Waits: Explicit waits are a crucial element of Selenium testing. They allow your tests to pause until a specific condition is met, preventing timing-related issues and ensuring a smoother test execution. These waits help synchronize your test script with the web application.
- Embrace Reusable Code: Reusable code is a best practice that can significantly enhance the efficiency and maintainability of your automation projects. It enables you to write code once and reuse it across multiple test cases, reducing duplication and simplifying updates.
- Leverage CSS Selectors: CSS selectors are powerful tools for locating and interacting with web page elements in Selenium. They offer flexibility in selecting elements based on attributes and properties, making it easier to target specific elements like buttons, links, and text boxes.
- Implement the Page Object Model (POM): POM is a widely adopted design pattern in Selenium testing. It separates test cases from UI details, improving code organization, reusability, and readability. Updates to UI elements can be quickly made in the corresponding page class.
- Practice Data-Driven Testing: Data-driven testing lets you execute the same test script with various input data sets. This technique is invaluable for testing applications under different scenarios, ensuring stability and reliability while saving time in script creation and maintenance.
- Embrace Parallel Testing: Parallel testing runs multiple test cases concurrently on different browsers, operating systems, or devices. It dramatically reduces execution time and enhances testing efficiency.
- Explore Headless Browsers: Headless browsers are a fantastic option for running Selenium tests efficiently without a graphical interface. They operate in the background and can be deployed on remote servers, offering faster execution times, increased stability, and reduced resource usage.
- Implement a Robust Reporting Framework: A strong reporting framework is essential for test success. It provides a comprehensive view of the test execution process, highlights areas needing attention, and delivers valuable insights to stakeholders. It helps track progress, identify bugs, and monitor application health.
- Adhere to Good Naming Conventions: Employing meaningful names for elements, methods, and variables in your Selenium test code is vital for readability and maintainability. Descriptive and clear names should accurately represent their purpose and functionality.
- Leverage Source Control: Source control is a fundamental component of Selenium automation testing that facilitates collaboration, version control, and tracking changes in test scripts. It enables developers and testers to work together, manage code versions, and resolve conflicts efficiently.
- Implement Assertions: Assertions are essential for validating the correctness of the application under test. They help ensure that expected behavior aligns with actual behavior during test execution, aiding in early defect detection.
- Utilize Test Coverage Tools: Test coverage tools are valuable in assessing the effectiveness of your testing efforts. They reveal gaps in test coverage by measuring the percentage of code executed during testing, enhancing code quality.
- Implement Error Handling: Effective error handling is crucial to maintaining the stability and reliability of your test automation framework. Anticipating and handling potential errors during test execution prevents unexpected failures and provides informative feedback.
- Conduct Code Reviews: Code reviews are critical for Selenium automation testing success. They involve systematic examinations of code to ensure it meets quality standards, leading to improved code quality, defect identification, and reduced maintenance costs.
- Prioritize Tests: Test prioritization enhances testing efficiency by executing critical tests first. It helps identify and resolve critical issues quickly, saving time and resources.
- Adopt Test Automation Frameworks: Test automation frameworks provide structure for writing, organizing, and executing automated tests. They enable efficient test script development, consistency, and robust reporting and analysis capabilities.
- Manage Test Data: Proper test data management ensures test reliability and accuracy. Managing and maintaining test data, including login credentials and input values, prevents test failures caused by incorrect or outdated data.
- Utilize Debugging Tools: Debugging tools are indispensable for identifying and resolving code issues in Selenium automation testing. Integrated Development Environments (IDEs) offer debugging functionalities like breakpoints and step-by-step execution.
- Implement Continuous Integration (CI): Continuous Integration practices involve integrating code changes regularly, and automatically building and testing integrated code. CI streamlines the testing process and detects issues early in the development cycle.
- Incorporate Performance Testing: Performance testing assesses how well an application performs under specific conditions, measuring response time, throughput, and resource utilization. It helps identify scalability issues and performance bottlenecks.
By incorporating these practices into your Selenium automation testing, you can streamline your testing process, improve test coverage, and deliver more reliable and efficient test results.
To Sum It Up
Selenium is indeed an outstanding tool for automating your web application testing requirements. However, like any platform, there are some valuable best practices and tips that can help you maximize the benefits of using Selenium.
By implementing the techniques mentioned above, you can simplify your Selenium tests, enhance their organization, and save valuable time. Additionally, when selecting a Selenium-based platform for your testing needs, it’s essential to choose one that streamlines the automated testing process. Keep an eye out for tools that offer testing on a real device cloud, such as LambdaTest. LambdaTest is thoughtfully designed to make life easier for testers by providing developer-friendly resources like built-in development tools and seamless integrations with popular programming languages and frameworks. As a result, it accelerates and streamlines automated Selenium testing, yielding faster, smoother, and more effective results.
Incorporating these practices and leveraging tools like LambdaTest can significantly improve the efficiency and effectiveness of your Selenium automation testing efforts, ensuring your web applications are thoroughly tested and ready to provide an exceptional user experience.